API Documentation
Basics
The auth_token required to access the API is a 36 digit UUID.
All API access must be over HTTPS. POST requests must include your account name and secure auth token in the header and be posted to https://api.bramble.io/[endpoint]
. The API responds with a JSON object detailing success or failure of the request. In the case of failure, a reason is returned.
Normally this will be initiated & handled via your enterprise platform, but can be tested from the command line using curl
curl -X POST https://api.bramble.io/PING \
-H 'agency:yourAgency' \
-H 'auth_token:yourToken'
https.request({
host : "api.bramble.io",
path : "/PING",
method : "POST",
headers : {
"agency": yourAgency
"auth_token": yourToken
}
},
function(response) {
// Do awesome stuff with Bramble data!
})
{
"APImethod": "PING",
"status": "success",
"result": "apiPONG"
}
To get an up to date list of the commands accessible to your account use /getPermissions
curl -X POST https://api.bramble.io/getPermissions \
-H 'agency:yourAgency' \
-H 'auth_token:yourToken'
https.request({
host : "api.bramble.io",
path : "/getPermissions",
method : "POST",
headers : {
"agency": yourAgency
"auth_token": yourToken
}
},
function(response) {
// With great power comes great responsibility!
})
{
"APImethod": "getPermissions",
"status": "success",
"permissions": [
"PING",
"sessionPING",
"getStats",
"toastMSG",
"shareURL",
"pollySession",
"createRoom"
]
}
Rooms & Users
For manageable numbers you will normally invite tutors to create their own rooms using the inviteTutors page provided for your account. This gives tutors choice over their room name and exposes them to the tutorial flow. As your volume grows you may prefer to integrate this programatically into your back end. You can create rooms directly via the API endpoint /createRoom
. These will sit within your subdomain, and all usage will show up for analysis in your reporting.
{
"agency": yourAgency,
"auth_token": yourToken,
"room": "JaneBloggs"
}
{
"APImethod": "createRoom",
"status": "success",
"result": "https://yourAgency.bramble.io/JaneBloggs"
}
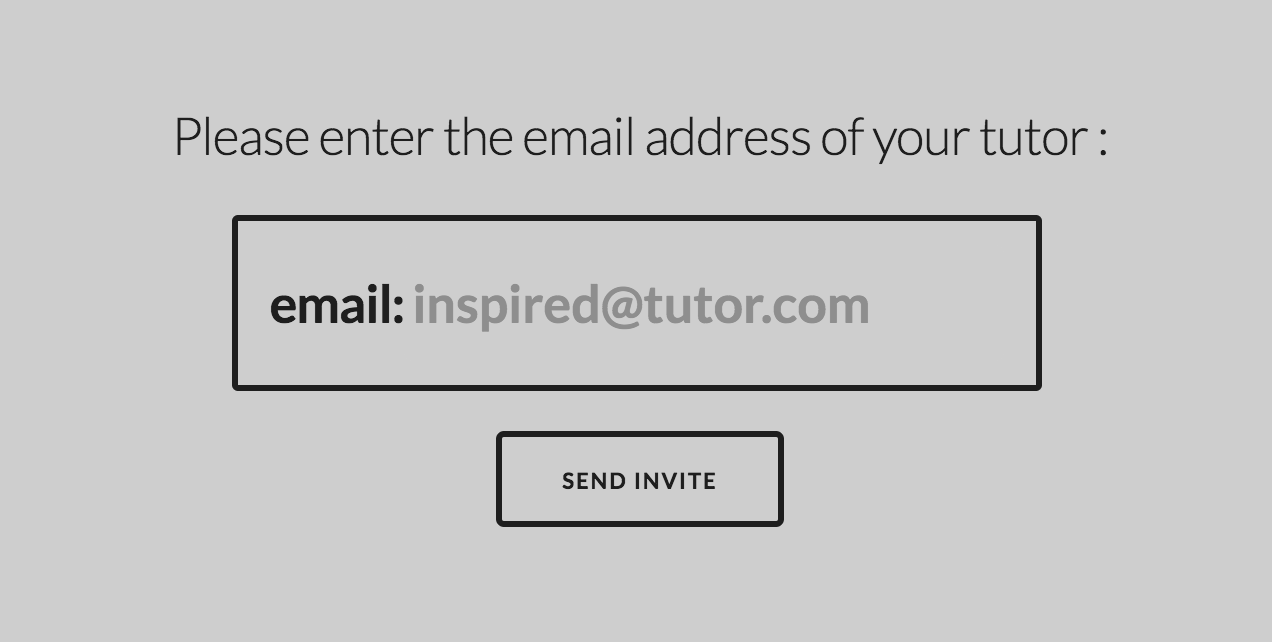